1. Read-Only (Simple) Synchronization
Description:
This pattern is designed for scenarios where data is static and does not require frequent updates. It ensures that the entire dataset is synchronized from the server to the local storage in one go. This approach is ideal for reference data or lookup tables that rarely change, minimizing complexity in synchronization logic.
Implementation Steps:
- Fetch All Data: Retrieve the entire dataset from the server to local storage without applying filters.
Replace Existing Data: Clear local storage and replace it with the newly fetched data to ensure consistency
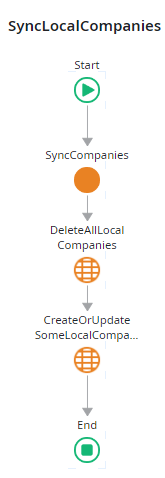
Filter Data for Relevance: Ensure only the minimum subset of relevant data is stored to optimize performance and reduce storage usage.
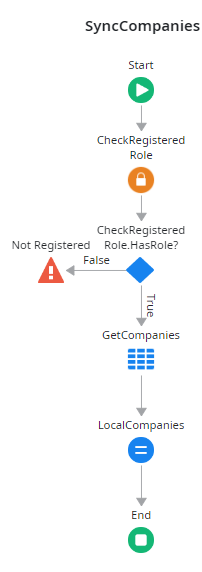
Pros:
- Simple Logic: Easy to implement without complex tracking mechanisms.
- Consistent Data: Ensures local storage always contains the latest data from the server.
- Ideal for Small Data Sets: Suitable for static or reference data like product categories or country lists.
Cons:
- High Data Transfer: Full data replacement may result in larger data synchronization.
- Slower for Large Data Sets: Full synchronization duration can increase with bigger datasets.
- No Incremental Updates: Always replaces the entire dataset, even if only a few records have changed.
2. Read-Only Data Synchronization
Description:
This pattern is designed for scenarios where data is frequently updated but should only be accessed and viewed by users without allowing modifications on the device. It ensures that only the necessary data is transferred, thereby saving bandwidth and optimizing the synchronization process.
Implementation Steps:
- Track Changes: Use a ModifiedOn timestamp to track when a record was last updated on the server.
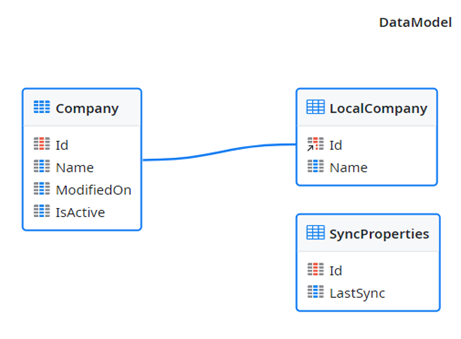
- Create Sync Logic: Implement logic that only fetches records modified since the last sync. This can be done by comparing the last sync timestamp stored in the app with the ModifiedOn timestamp of the records.
- Track Deleted Records: Use the IsActive attribute to identify and track deleted records.
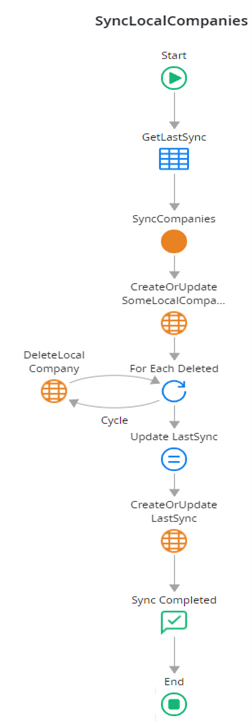
- Sync Data: Retrieve the list of changed, added, and deleted records. Update or delete records in the local storage accordingly.
- Store Sync Properties: Update the SyncProperties.LastSync entity with the latest synchronization timestamp returned by the server to keep track of sync history.
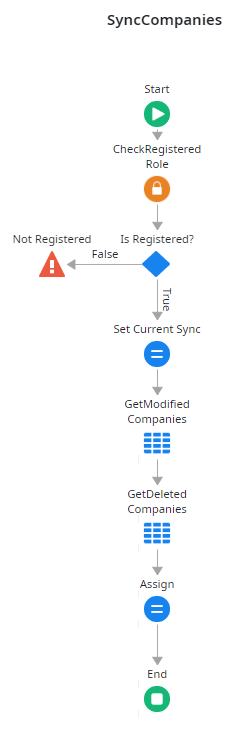
Pros:
- Bandwidth Saving: Only modified records are synced, reducing unnecessary data transfer.
- Faster Sync: Incremental updates speed up synchronization times compared to full data refreshes.
Cons:
- Tracking Changes: Requires additional logic to track changes and deletions on the server and manage timestamps for each record.
- Limited Data Availability: Only updated data is synced; initial data loading could be time-consuming.
3. Read/Write Data Synchronization (Last Write Wins)
Description:
In this pattern, the most recent modification made to a record on the device is the one that prevails when synchronizing with the server. This pattern is best suited for scenarios where data conflicts are rare and the application can tolerate overwriting old data.
Implementation Steps:
- Create Local Entity: Mirror the server data entity locally on the device (e.g., create LocalCompany for Company).
Generate Sync Actions: Use Service Studio to generate synchronization actions that will sync the local data with the server
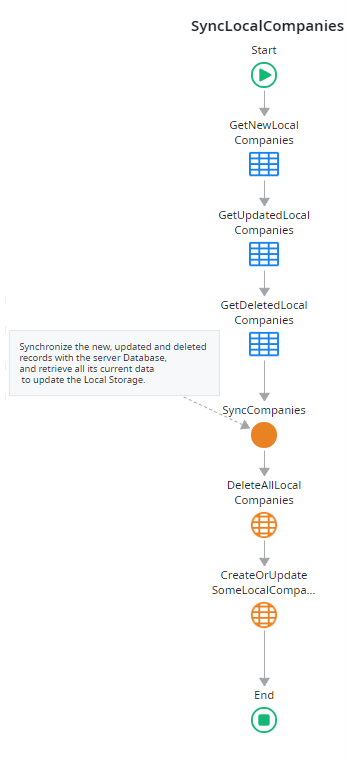
- Track Modifications: Detect locally modified records (using IsModified flags or similar) and push them to the server.
- Sync Changes: Sync changes with the server by overwriting older records with the latest modifications.
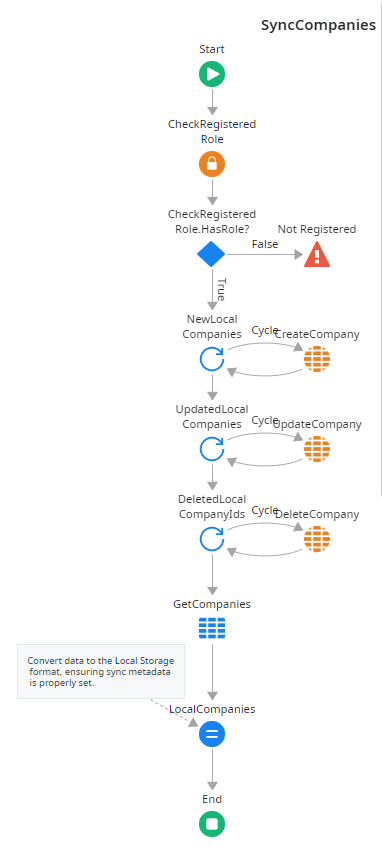
Pros:
- Simple to Implement: Easy to set up, making it a great choice for small apps with limited data.
- Ideal for Non-Critical Data: Perfect for use cases like user preferences where the most recent update is always the desired one.
Cons:
- Risk of Data Loss: If multiple users update the same record at the same time, the last update will overwrite all previous changes, leading to potential data loss.
- Not Suitable for Critical Data: For use cases that require data consistency, this pattern is not recommended.
4. Read/Write Data Synchronization with Conflict Detection
Description:
This pattern is best for scenarios where multiple users may be editing the same data offline, and conflicts are likely to occur. It allows conflicts to be detected and flagged for manual or automatic resolution, ensuring data consistency.
Implementation Steps:
- Create Local Entity: Mirror the server data entity locally on the device and include metadata attributes such as Sync Status and Sync Time stamp to track synchronization status.
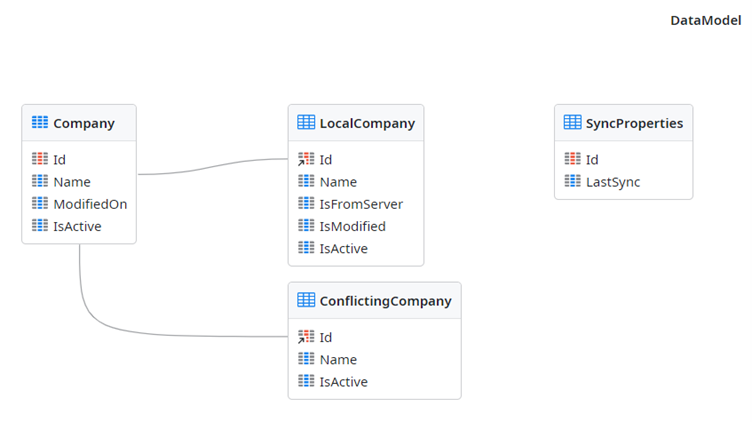
- Generate Sync Actions: Automatically generate sync actions using Service Studio to handle data transfer between the server and local storage.
- Detect Changes: When syncing, detect conflicts by comparing the local changes with the server’s most recent data.
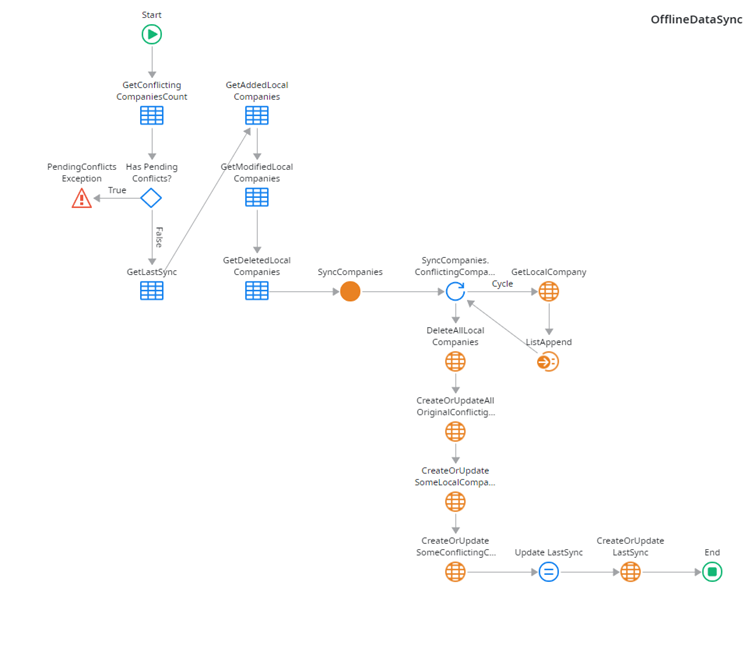
- Resolve Conflicts: Handle conflicts manually or automatically. For manual resolution, flag the conflicting records for the user to resolve. For automatic resolution, define rules (e.g., the most recent update wins) to resolve the conflicts.
- Sync Resolved Data: After conflicts are resolved, push the changes back to the server.
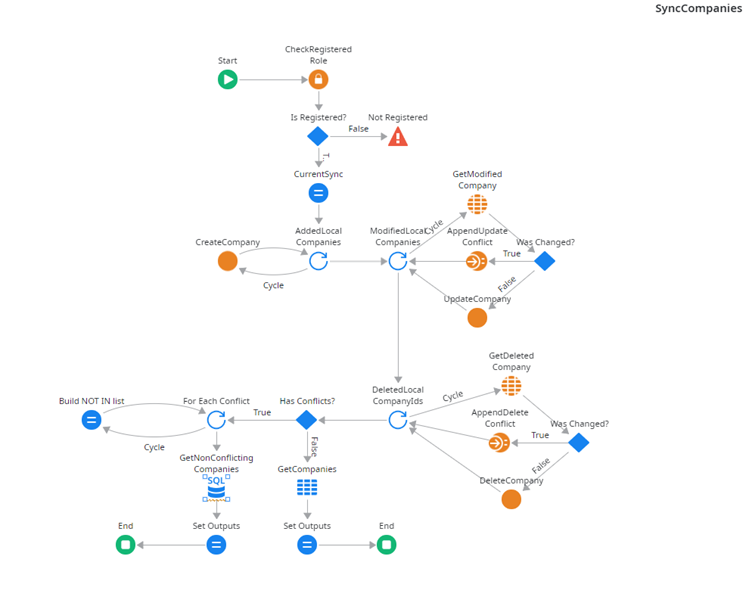
Pros:
- Handles Simultaneous Updates: Works well for applications where multiple users may update the same data at the same time.
- Conflict Detection: Allows the system to detect and resolve conflicts, ensuring consistency.
Cons:
- Complexity: Implementing conflict detection and resolution adds significant complexity to the synchronization logic.
- Performance: Resolving conflicts can impact performance, especially with large datasets.
5. Read/Write Data One-to-Many Synchronization
Description:
This pattern is ideal for handling hierarchical or parent-child relationships between data entities. It ensures that both the parent and child records are synchronized correctly, preserving relationships and ensuring data consistency across the application.
Implementation Steps:
- Define Local Entities: Define entities for both the parent and child records in the local storage.
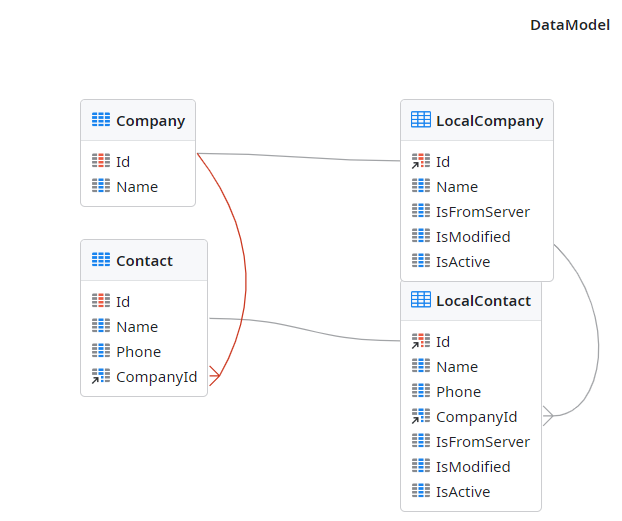
- Add Metadata Attributes: Add attributes like IsFromServer, IsModified, and IsActive to help manage synchronization status.
- Implement Sync Logic: Ensure that the synchronization logic properly handles the relationships between parent and child records. This may involve ensuring that changes in a parent record trigger updates to associated child records.
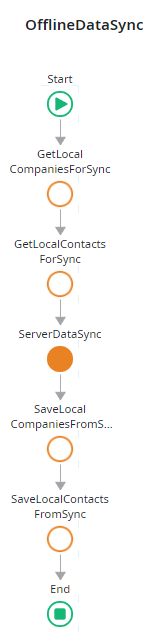
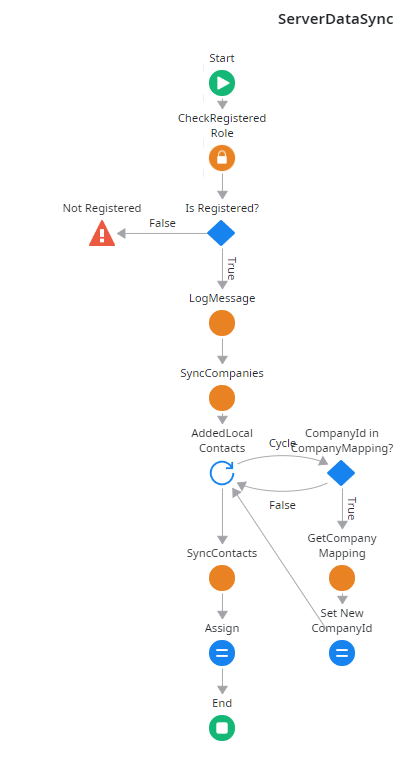
- Batch Synchronization: Implement logic to handle batch synchronization, ensuring that both parent and child records are synced together as a batch to preserve their relationships.
- Sync Data: When syncing, update both parent and child records, ensuring that any changes to child records are properly synced with the server and vice versa.
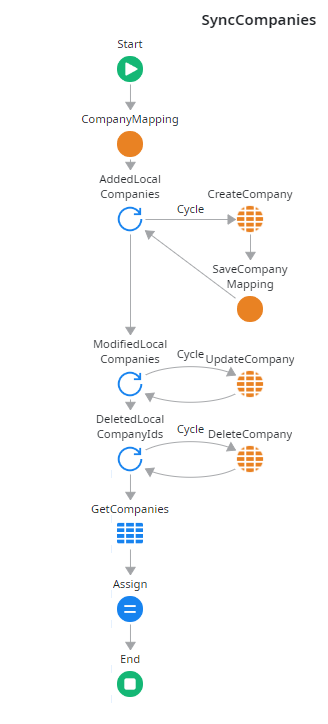
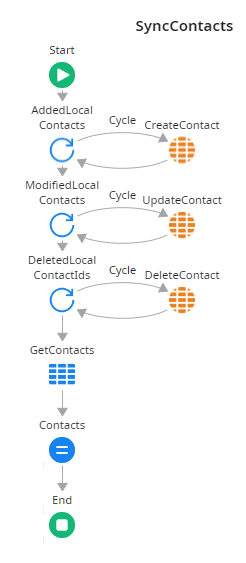
Pros:
- Ideal for Hierarchical Data: Perfect for applications that involve parent-child relationships, such as inventory systems or organizational structures.
- Efficient Bulk Updates: Batch synchronization can handle large volumes of data efficiently.
Cons:
- Complexity: Managing relationships between parent and child records requires careful handling to avoid data inconsistencies.
- Resource-Intensive: Synchronizing large datasets with multiple layers of data (parent-child) can be resource-intensive and may require optimization techniques.